Product Promotion
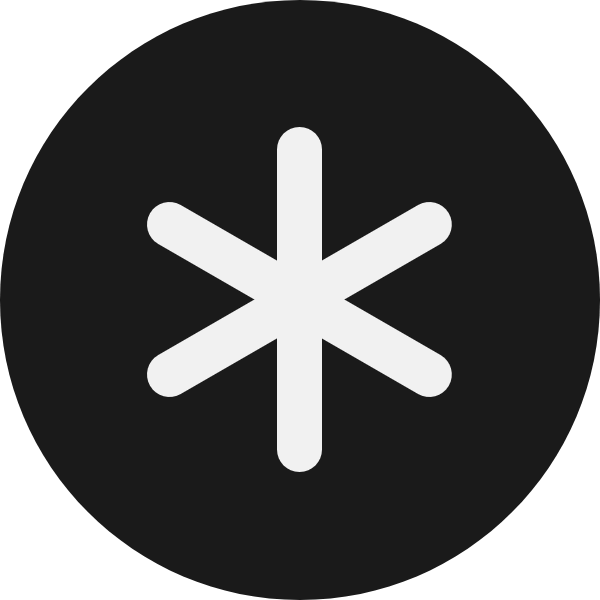
0x5a.live
for different kinds of informations and explorations.
GitHub - KipData/FnckSQL: SQL as a Function for Rust
SQL as a Function for Rust. Contribute to KipData/FnckSQL development by creating an account on GitHub.
Visit SiteGitHub - KipData/FnckSQL: SQL as a Function for Rust
SQL as a Function for Rust. Contribute to KipData/FnckSQL development by creating an account on GitHub.
Powered by 0x5a.live 💗
What is FnckSQL
FnckSQL is a high-performance SQL database that can be embedded in Rust code (based on RocksDB by default), making it possible to call SQL just like calling a function. It supports most of the syntax of SQL 2016.
Quick Started
Tips: Install rust toolchain and llvm first.
Clone the repository
git clone https://github.com/KipData/FnckSQL.git
Using FnckSQL in code
let fnck_sql = DataBaseBuilder::path("./data").build()?;
let tuples = fnck_sql.run("select * from t1")?;
TPCC
run cargo run -p tpcc --release
to run tpcc
- i9-13900HX
- 32.0 GB
- YMTC PC411-1024GB-B
- Tips: TPCC currently only supports single thread
<90th Percentile RT (MaxRT)>
New-Order : 0.005 (0.007)
Payment : 0.084 (0.141)
Order-Status : 0.492 (0.575)
Delivery : 6.109 (6.473)
Stock-Level : 0.001 (0.001)
<TpmC>
89.9205557572134 Tpmc
PG Wire Service
run cargo run --features="net"
to start server
then use
psql
to enter sql
Storage Support:
- RocksDB
Docker
Pull Image
docker pull kould23333/fncksql:latest
Build From Source
git clone https://github.com/KipData/FnckSQL.git
cd FnckSQL
docker build -t kould23333/fncksql:latest .
Run
We installed the psql
tool in the image for easy debug.
You can use psql -h 127.0.0.1 -p 5432
to do this.
docker run -d \
--name=fncksql \
-p 5432:5432 \
--restart=always \
-v fncksql-data:/fnck_sql/fncksql_data \
-v /etc/localtime:/etc/localtime:ro \
kould23333/fncksql:latest
Features
- ORM Mapping:
features = ["macros"]
#[derive(Default, Debug, PartialEq)]
struct MyStruct {
c1: i32,
c2: String,
}
implement_from_tuple!(
MyStruct, (
c1: i32 => |inner: &mut MyStruct, value| {
if let DataValue::Int32(Some(val)) = value {
inner.c1 = val;
}
},
c2: String => |inner: &mut MyStruct, value| {
if let DataValue::Utf8(Some(val)) = value {
inner.c2 = val;
}
}
)
);
- User-Defined Function:
features = ["macros"]
scala_function!(TestFunction::test(LogicalType::Integer, LogicalType::Integer) -> LogicalType::Integer => |v1: DataValue, v2: DataValue| {
let plus_binary_evaluator = EvaluatorFactory::binary_create(LogicalType::Integer, BinaryOperator::Plus)?;
let value = plus_binary_evaluator.binary_eval(&v1, &v2);
let plus_unary_evaluator = EvaluatorFactory::unary_create(LogicalType::Integer, UnaryOperator::Minus)?;
Ok(plus_unary_evaluator.unary_eval(&value))
});
let fnck_sql = DataBaseBuilder::path("./data")
.register_scala_function(TestFunction::new())
.build()?;
- User-Defined Table Function:
features = ["macros"]
table_function!(MyTableFunction::test_numbers(LogicalType::Integer) -> [c1: LogicalType::Integer, c2: LogicalType::Integer] => (|v1: DataValue| {
let num = v1.i32().unwrap();
Ok(Box::new((0..num)
.into_iter()
.map(|i| Ok(Tuple {
id: None,
values: vec![
DataValue::Int32(Some(i)),
DataValue::Int32(Some(i)),
]
}))) as Box<dyn Iterator<Item = Result<Tuple, DatabaseError>>>)
}));
let fnck_sql = DataBaseBuilder::path("./data")
.register_table_function(MyTableFunction::new())
.build()?;
- Optimizer
- RBO
- CBO based on RBO(Physical Selection)
- Execute
- Volcano
- MVCC Transaction
- Optimistic
- Field options
- [not] null
- unique
- primary key
- SQL where options
- is [not] null
- [not] like
- [not] in
- Supports index type
- PrimaryKey
- Unique
- Normal
- Composite
- Supports multiple primary key types
- Tinyint
- UTinyint
- Smallint
- USmallint
- Integer
- UInteger
- Bigint
- UBigint
- Char
- Varchar
- DDL
- Begin (Server only)
- Commit (Server only)
- Rollback (Server only)
- Create
- Table
- Index: Unique\Normal\Composite
- View
- Drop
- Table
- Index
- View
- Alert
- Add Column
- Drop Column
- Truncate
- DQL
- Select
- SeqScan
- IndexScan
- Where
- Distinct
- Alias
- Aggregation: count()/sum()/avg()/min()/max()
- SubQuery[select/from/where]
- Join: Inner/Left/Right/Full/Cross (Natural\Using)
- Group By
- Having
- Order By
- Limit
- Show Tables
- Explain
- Describe
- Union
- Select
- DML
- Insert
- Insert Overwrite
- Update
- Delete
- Analyze
- Copy To
- Copy From
- DataTypes
- Invalid
- SqlNull
- Boolean
- Tinyint
- UTinyint
- Smallint
- USmallint
- Integer
- UInteger
- Bigint
- UBigint
- Float
- Double
- Char
- Varchar
- Date
- DateTime
- Time
- Tuple
Roadmap
- SQL 2016
License
FnckSQL uses the Apache 2.0 license to strike a balance between open contributions and allowing you to use the software however you want.
Contributors
Thanks For
- Fedomn/sqlrs: Main reference materials, Optimizer and Executor all refer to the design of sqlrs
- systemxlabs/bustubx
- duckdb/duckdb
Rust Resources
are all listed below.
Made with ❤️
to provide different kinds of informations and resources.