Product Promotion
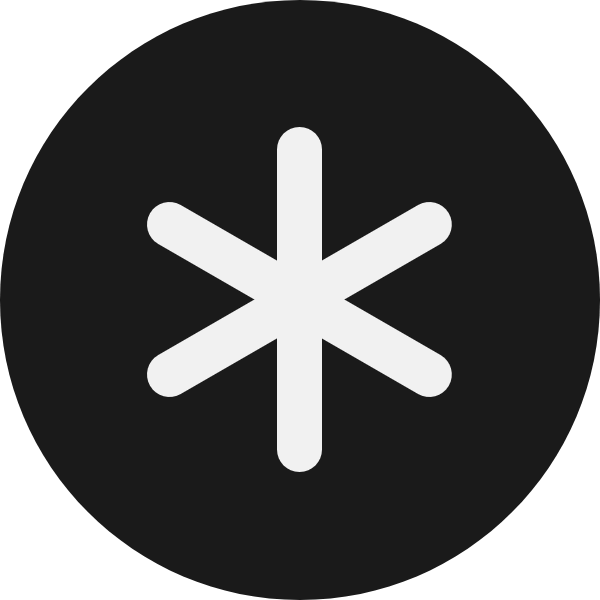
0x5a.live
for different kinds of informations and explorations.
GitHub - h2non/semver.c: Semantic version in ANSI C
Semantic version in ANSI C. Contribute to h2non/semver.c development by creating an account on GitHub.
Visit SiteGitHub - h2non/semver.c: Semantic version in ANSI C
Semantic version in ANSI C. Contribute to h2non/semver.c development by creating an account on GitHub.
Powered by 0x5a.live 💗
semver.c

Semantic version v2.0 parser and render written in ANSI C with zero dependencies.
Features
- Standard compliant (otherwise, open an issue)
- Version metadata parsing
- Version prerelease parsing
- Version comparison helpers
- Supports comparison operators
- Version render
- Version bump
- Version sanitizer
- 100% test coverage
- No regexp (ANSI C doesn't support it)
- Numeric conversion for sorting/filtering
Versions
Usage
Basic comparison:
#include <stdio.h>
#include <semver.h>
char current[] = "1.5.10";
char compare[] = "2.3.0";
int
main(int argc, char *argv[]) {
semver_t current_version = {};
semver_t compare_version = {};
if (semver_parse(current, ¤t_version)
|| semver_parse(compare, &compare_version)) {
fprintf(stderr,"Invalid semver string\n");
return -1;
}
int resolution = semver_compare(compare_version, current_version);
if (resolution == 0) {
printf("Versions %s is equal to: %s\n", compare, current);
}
else if (resolution == -1) {
printf("Version %s is lower than: %s\n", compare, current);
}
else {
printf("Version %s is higher than: %s\n", compare, current);
}
// Free allocated memory when we're done
semver_free(¤t_version);
semver_free(&compare_version);
return 0;
}
Satisfies version:
#include <stdio.h>
#include <semver.h>
semver_t current = {};
semver_t compare = {};
int
main(int argc, char *argv[]) {
semver_parse("1.3.10", ¤t);
semver_parse("1.5.2", &compare);
// Use caret operator for the comparison
char operator[] = "^";
if (semver_satisfies(current, compare, operator)) {
printf("Version %s can be satisfied by %s", "1.3.10", "1.5.2");
}
// Free allocated memory when we're done
semver_free(¤t);
semver_free(&compare);
return 0;
}
Installation
Clone this repository:
$ git clone https://github.com/h2non/semver.c
Or install with clib:
$ clib install h2non/semver.c
API
struct semver_t { int major, int minor, int patch, char * prerelease, char * metadata }
semver base struct.
semver_parse(const char *str, semver_t *ver) => int
Parses a string as semver expression.
Returns:
-1
- In case of invalid semver or parsing error.0
- All was fine!
semver_compare(semver_t a, semver_t b) => int
Compare versions a
with b
.
Returns:
-1
in case of lower version.0
in case of equal versions.1
in case of higher version.
semver_satisfies(semver_t a, semver_t b, char *operator) => int
Checks if both versions can be satisfied based on the given comparison operator.
Allowed operators:
=
- Equality>=
- Higher or equal to<=
- Lower or equal to<
- Lower than>
- Higher than^
- Caret operator comparison (more info)~
- Tilde operator comparison (more info)
Returns:
1
- Can be satisfied0
- Cannot be satisfied
semver_satisfies_caret(semver_t a, semver_t b) => int
Checks if version x
can be satisfied by y
performing a comparison with caret operator.
See: https://docs.npmjs.com/misc/semver#caret-ranges-1-2-3-0-2-5-0-0-4
Returns:
1
- Can be satisfied0
- Cannot be satisfied
semver_satisfies_patch(semver_t a, semver_t b) => int
Checks if version x
can be satisfied by y
performing a comparison with tilde operator.
See: https://docs.npmjs.com/misc/semver#tilde-ranges-1-2-3-1-2-1
Returns:
1
- Can be satisfied0
- Cannot be satisfied
semver_eq(semver_t a, semver_t b) => int
Equality comparison.
semver_ne(semver_t a, semver_t b) => int
Non equal comparison.
semver_gt(semver_t a, semver_t b) => int
Greater than comparison.
semver_lt(semver_t a, semver_t b) => int
Lower than comparison.
semver_gte(semver_t a, semver_t b) => int
Greater than or equal comparison.
semver_lte(semver_t a, semver_t b) => int
Lower than or equal comparison.
semver_render(semver_t *v, char *dest) => void
Render as string.
semver_numeric(semver_t *v) => int
Render as numeric value. Useful for ordering and filtering.
semver_bump(semver_t *a) => void
Bump major version.
semver_bump_minor(semver_t *a) => void
Bump minor version.
semver_bump_patch(semver_t *a) => void
Bump patch version.
semver_free(semver_t *a) => void
Helper to free allocated memory from heap.
semver_is_valid(char *str) => int
Checks if the given string is a valid semver expression.
semver_clean(char *str) => int
Removes invalid semver characters in a given string.
License
MIT - Tomas Aparicio
C++ Programming Resources
are all listed below.
Made with ❤️
to provide different kinds of informations and resources.